Moving our game logic to an app
Since my game was already programmed in Python, it was only natural that the playable application part of it be programmed in Python as well. And so I decided to give the python library Kivy a try.
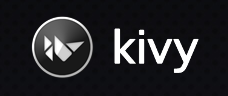
Not a fan of Kivy
Okay, I admit, I really did give it a try for a solid week. I really did. I even wanted to throw a few bucks the developer's way to support the library. I get that there's always a period of getting used to a library, but Kivy gave me a very unpleasant experience from start to finish. It was so unpleasant that I deemed it faster for me to completely scrap Kivy after a week and rewrite everything in a completely different language. I condensed my review down to points listed below:
- Deploying to my phone was horrible. Absolutely horrible. It was a nightmare to set up and once I finally got it working, even compiling the sample code produced weird bugs on Android. At the time of this post, there's one or two aging issues against it in GitHub already.
- Performance on my Android phone was terrible. Granted, my phone has a couple years on it, but more impressive 3D games run 10x smoother than the tiny 2D example apps did on my phone.
- Building applications gives you a choice of KV files, hardcoding it in python, or loading strings of kv code into Kivy's builder. One of the first articles states kv files are preferred, but a lot of the API and examples intermix the other two. It's fairly easy to translate, so this was more of a minor annoyance than major bug.
- Scripts errors sometimes had segfault Kivy on Windows/Linux/Android. I've had small scripting errors (like loading a graphic file that doesn't exist) just flat out hard-crash Kivy instead of produce a runtime error. Since the normal feedback didn't exist, it made debugging even worse. The icing on the cake is when the exact same code file hard-crashes Kivy the first time and then runs fine subsequent times.
- The property system was an unexpected sticking point for me. Try passing anything that touches NumPy (which is what my project uses a lot of) and watch Kivy utterly destroy itself in it's confusion.
Rewriting the Game with Phaser
I've used this library in the past and had some great experiences with it. It's Javascript/TypeScript and deploys browser-based games. Using Cordova, we can even deploy applications to Android/iOS/etc. It took me about a day or three to get back up to speed and already I was further than I've gotten with Kivy in a week.
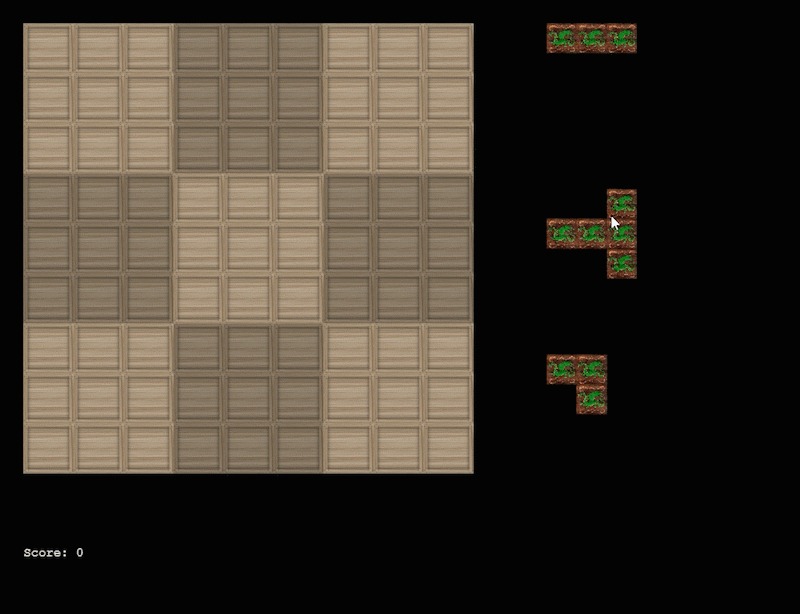
One can see that I've got most of the game functionality working already! One can place blocks, get points for clearing lines, and their block queue automatically refreshes!
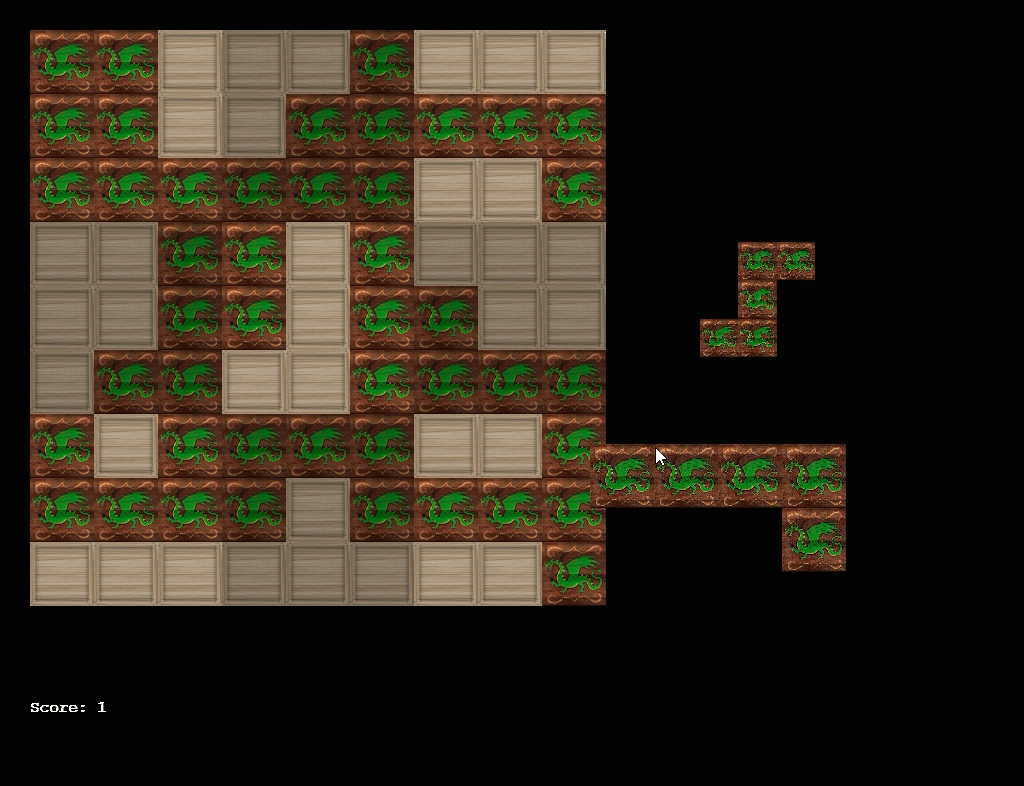
The code isn't too far off from the code seen in my previous post:
check_if_valid_move: function(x, y, block, board)
{
var newBoard = board;
var success = false;
// Bounds check
if(x < 0 || x > 9)
return {newBoard, success}
if(y < 0 || y > 9)
return {newBoard, success}
// Copy across board
testBoard = math.matrix(math.zeros(14,14)).subset(math.index(math.range(x,x+5), math.range(y,y+5)), block)
testBoard = math.add(board, testBoard)
if(math.max(testBoard) > 1)
return {newBoard, success};
newBoard = testBoard;
success = true;
return {newBoard, success};
}
Since I've only built this to establish a baseline score, there's no game over screens or anything like that. Since I'm pretty happy with how this is looking so far, I'll go ahead and see what sort of schools I can achieve in this game!
Baseline Results
Trial | Score |
---|---|
1 | 11 |
2 | 5 |
3 | 17 |
4 | 19 |
5 | 31 |
My score varies quite a bit, but averages out to about 17. That seems like a pretty good baseline to try to beat. Part 3 will start exploring the fun part of this project.. trying to solve the game!